Review A Repo's History
git log
- display all of the commits of a repository
- the SHA
- the author
- the date
- the message
- ...and more
git log --oneline
(use flag to alter display)
- lists one commit per line
- shows the first 7 characters of the commit's SHA
- shows the commit's message
git log --stat
- displays the file(s) that have been modified
- displays the number of lines that have been added/removed
- displays a summary line with the total number of modified files and lines that have been added/removed
command line pager
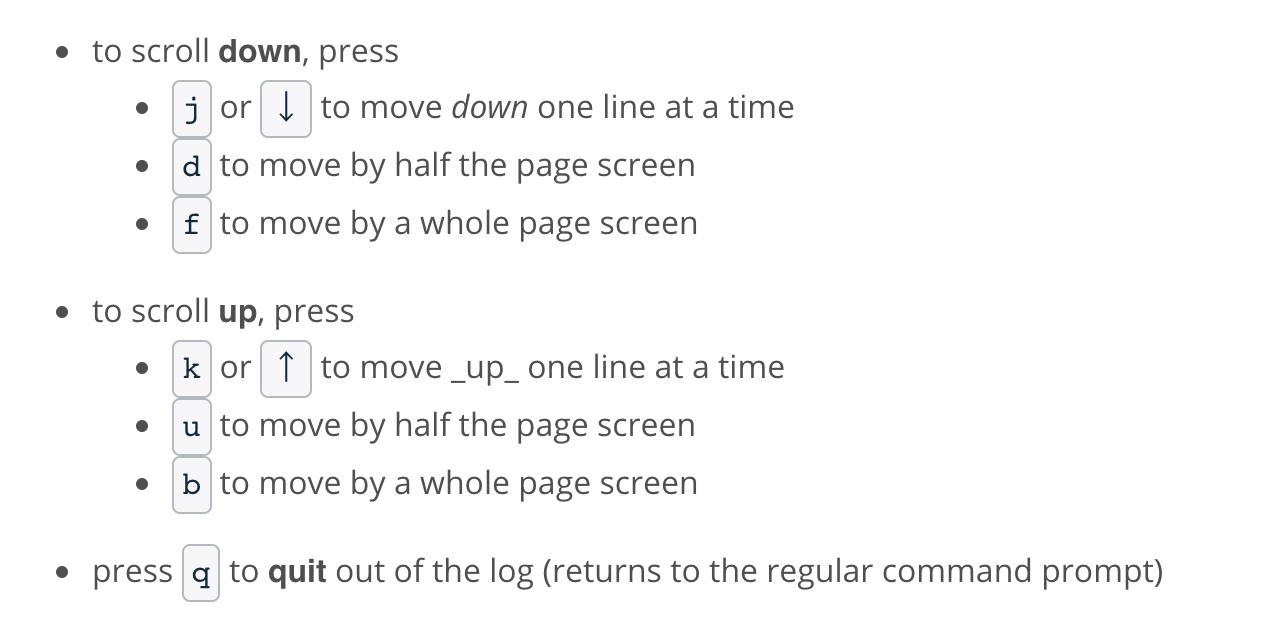
git log -p
(flag short for --patch)
git log -p -w
(-w flag ignores whitespace changes)
- displays the files that have been modified
- displays the location of the lines that have been added/removed
- displays the actual changes that have been made
git log -p SHA
OR git show -SHA
to look into a specific commit
Add Commits to A Repo
git add <file1> <file2>...<fileN>
- move files from the Working Directory to the Staging Index
git add .
refers to the all files and directories in current directory
git rm --cached
- removes file from the Staging Index
git commit -m "Initial commit"
- Bypass the editor with the
-m
flag
What to include in a Commit
- The goal is that each commit has a single focus
What makes good commit messages?
Do
- do keep the message short (less than 60-ish characters)
- do explain what the commit does (not how or why!)
Do not
- do not explain why the changes are made (more on this below)
- do not explain how the changes are made (that's what git log -p is for!)
- do not use the word "and"
What if you want to explain the Why
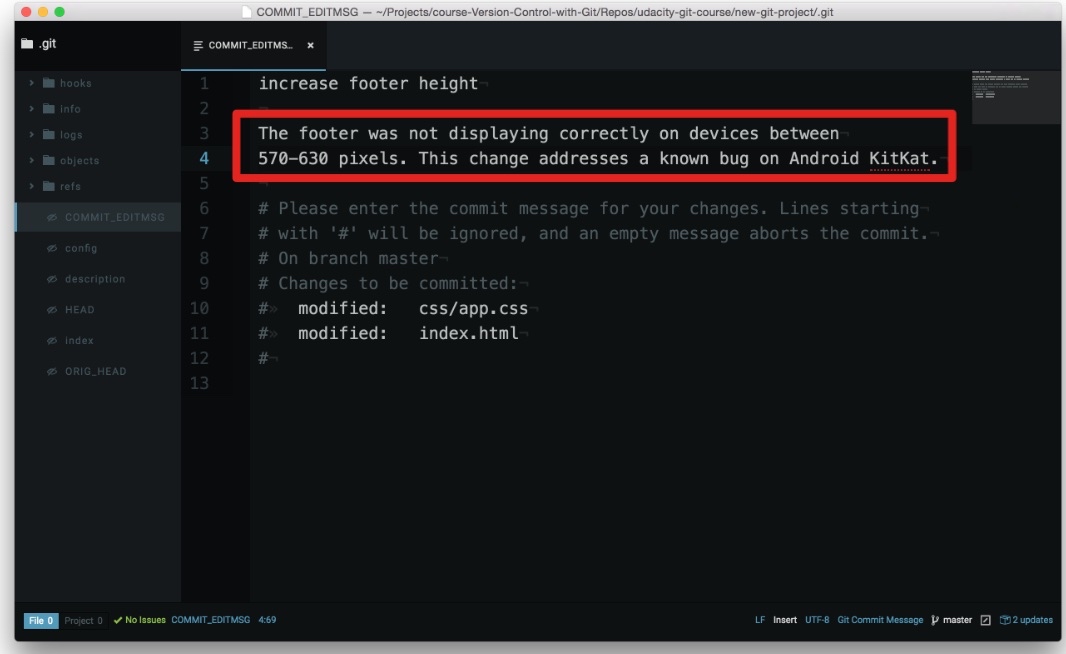
The information in red box is included in the git log
.
Udacity Git Commit Message Style Guide
git diff
- to see changes that have been made but haven't been committed, yet.
- the files that have been modified
- the location of the lines that have been added/removed
- the actual changes that have been made
- same as
git log -p
Having Git Ignore Files
- add
.gitignore
file to the same directory that the hidden .git
directory is located
What if there are a lot files to ignore?
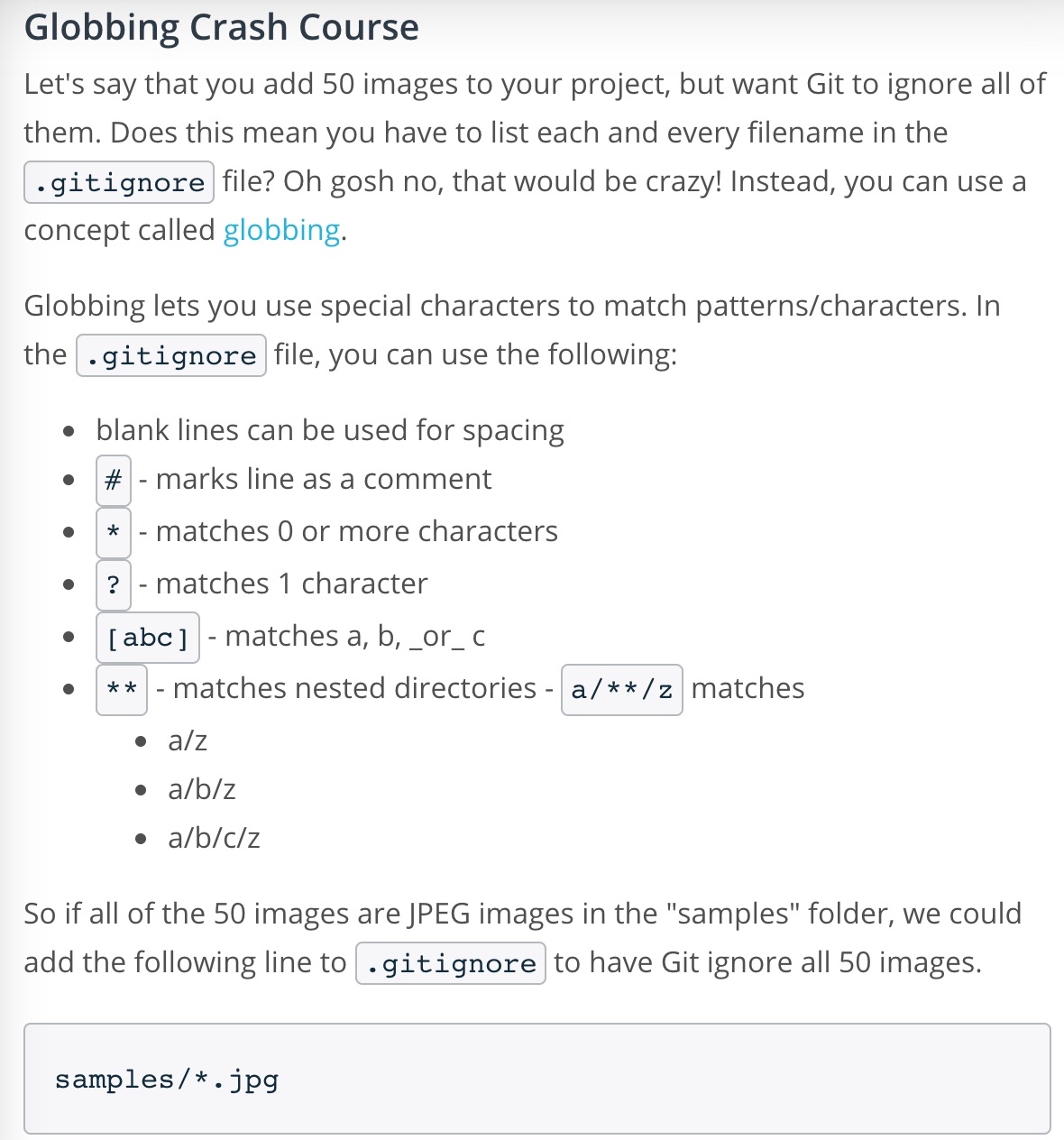
Tagging, Branching, and Merging
Tagging
git tag -a v1.0 (SHA)
- without providing SHA, git will tag the most recent commit
-a
flag (always use this flag) tells Git to create an annotated flag which include a lot of extra information such as:
- the person who made the tag
- the date the tag was made
- a message for the tag
- to delete a tag
git tag -d v1.0
Branching
Merging
- regular merge & fast-forward merge
- making a merge makes a commit
git merge <other-branch>
merge into the current 'checkout' branch
Merge Conflicts
- a merge conflict occurs when Git isn't sure which line(s) you want to use from the branches that are being merged.
- so we need to edit the same line on two different branches and then try to merge them.
- Steps to solve merge conflicts
- locate and remove all lines with merge conflict indicators
- determine what to keep
- save the file(s)
- stage the file(s)
- make a commit
Undoing Changes
Changing The Last Commit
git commit --amend
- If your Working Directory is clean, it will let you provide a new commit message.
- Add Forgotten Files (or changes to files) To Commit
- edit the file(s)
- save the file(s)
- stage the file(s)
- and run
git commit --amend
Reverting A Commit
git revert
git revert <SHA-of-commit-to-revert>
- will undo the changes that were made by the provided commit
- creates a new commit to record the change
Relative Commit References
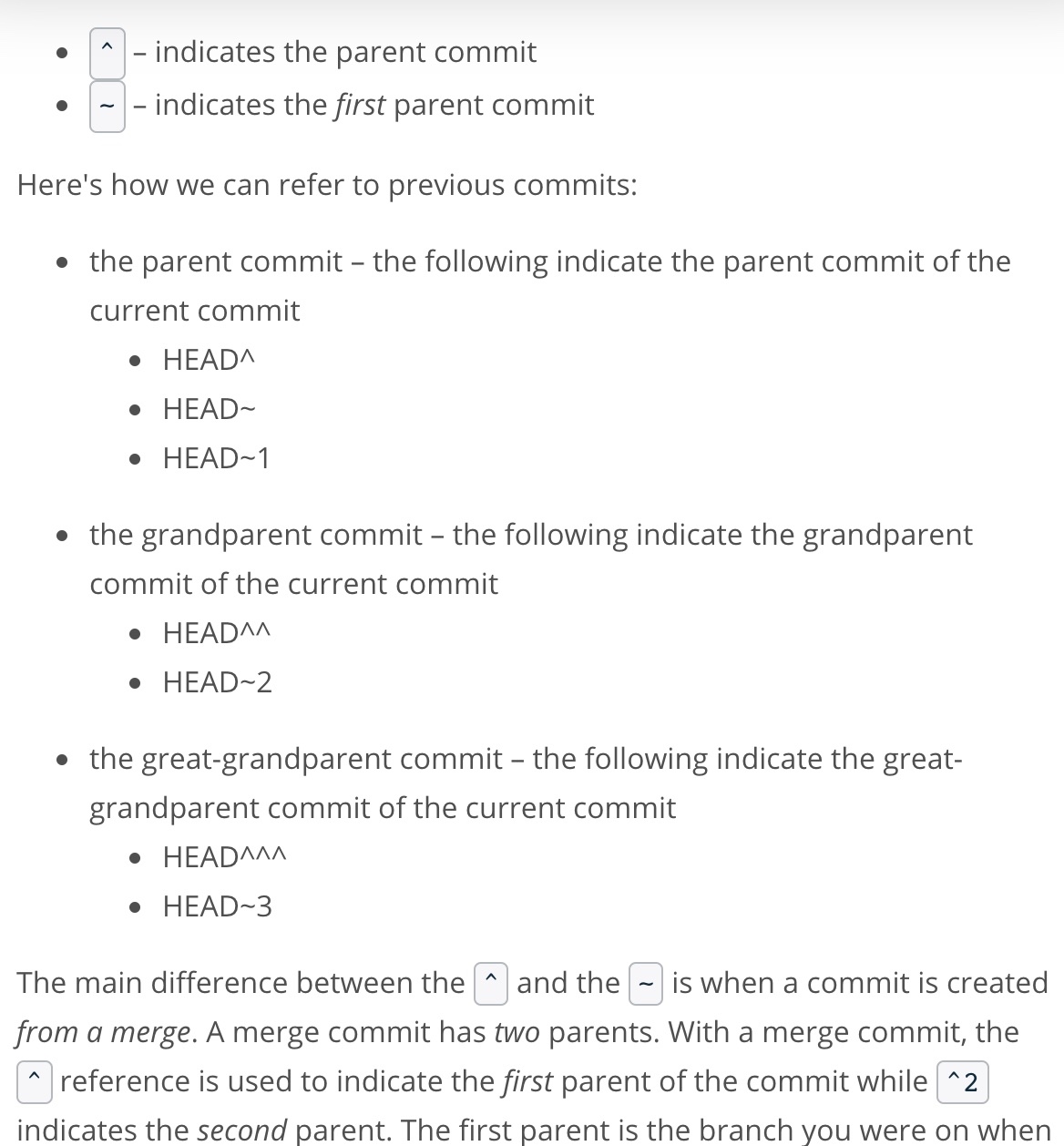
Resetting Commits
git reset <reference-to-commit>
- move the HEAD and current branch pointer to the referenced commit
- erase commits
- move committed changes to the staging index
- unstage committed changes
- flag --mixed (default without flag)
- before doing any resetting, create
backup
branch on the most-recent commit git branch backup
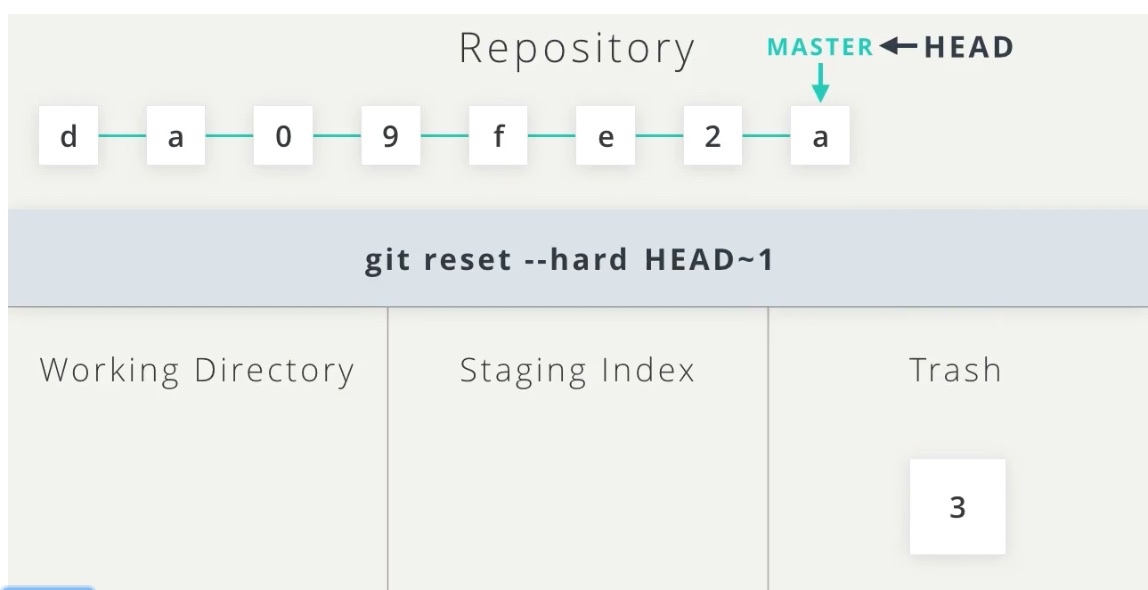
Back To Normal
- remove the uncommitted changes from the working directory
- git checkout -- index.html
- merge
backup
into master
(which will cause a Fast-forward merge and move master
up to the same point as backup
)
Remote Repositories
Add A Remote Repository
git remote add origin <url>
to set the shortname
git remote -v
to see the full path to the remote repository
Push Changes To A Remote
git push <remote-shortname> <branch>
Pulling Changes From A Remote
git pull <remote-shortname> <branch>
Pull vs Fetch
git pull
do two things:
- fetching remote changes (which adds the commits to the local repository and moves the tracking branch to point to them)
- merging the local branch with the tracking branch
- below is when we cannot use
git pull origin master
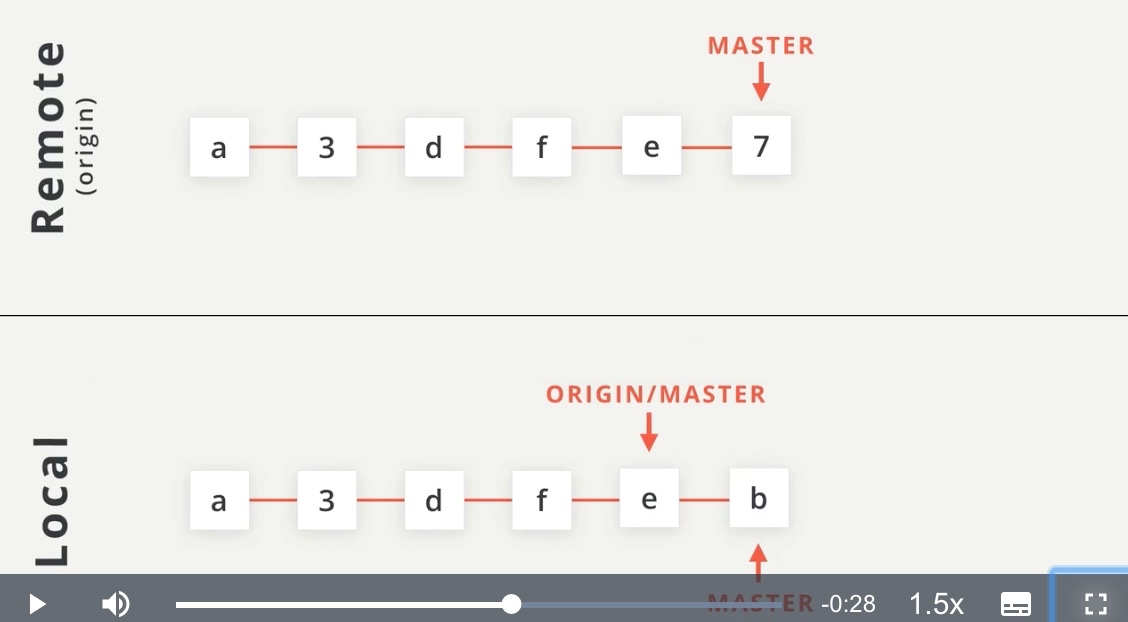
Working On Another Developer's Repository
Reviewing Existing Work
Filter Commits By Author
git shortlog -s -n
-s
to show just the number of commits
-n
to sort them numerically
git log
--author=surma
Filter Commits By Search
--grep
flag
git log --grep=bug
git log --grep="unit tests"
- Grep is a pattern matching tool
Determining What To Work On
- check
CONTRIBUTING.md
file
- GitHub Issues
Topic Branches
Update The README
Staying In Sync With A Remote Repository
Create a Pull Request
- A pull request is a request to the original or source repository's maintainer to include changes in their project that you made in your fork of their project. You are requesting that they pull in changes you've made.
Recap
A pull request is a request for the source repository to pull in your commits and merge them with their project. To create a pull request, a couple of things need to happen:
- you must fork the source repository
- clone your fork down to your machine
- make some commits (ideally on a topic branch!)
- push the commits back to your fork
- create a new pull request and choose the branch that has your new commits
Retrieving Upstream Changes
git fetch upstream master
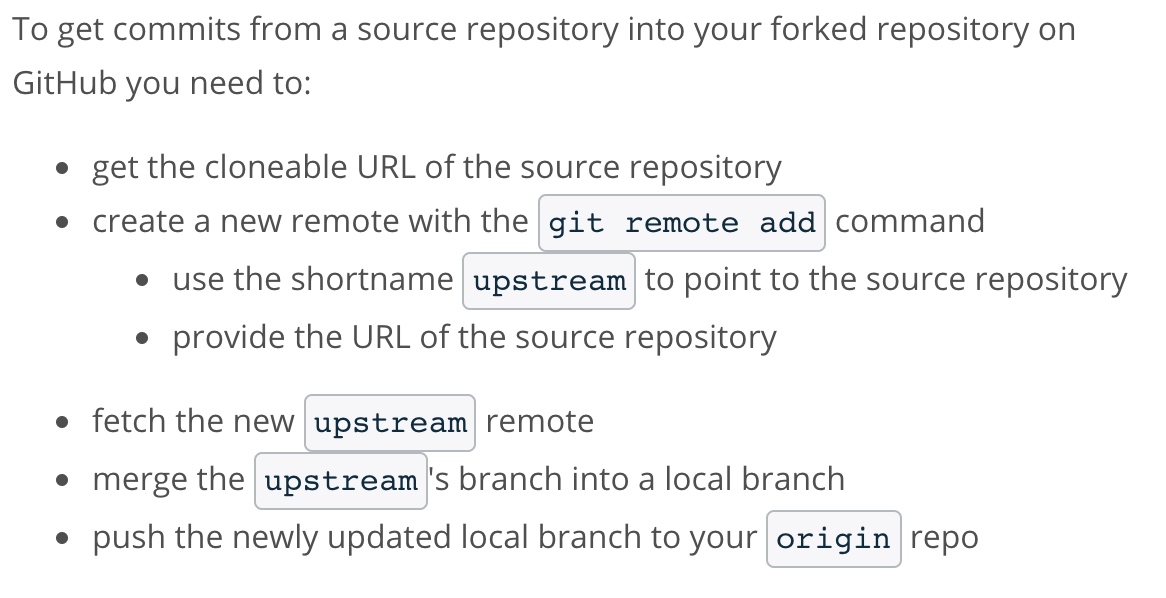
Manage an active PR
If the project's maintainer is requesting changes to the pull request, then:
- make any necessary commits on the same branch in your local repository that your pull request is based on
- push the branch to the your fork of the source repository
The commits will then show up on the pull request page.
Squash Commits
The Rebase Commandgit rebase -i HEAD~3
HEAD~3
as the base where all of the other commits (HEAD~2
, HEAD~1
, HEAD
) will connect to. (HEAD
point to current location)
-i
in the command stands for "interactive".
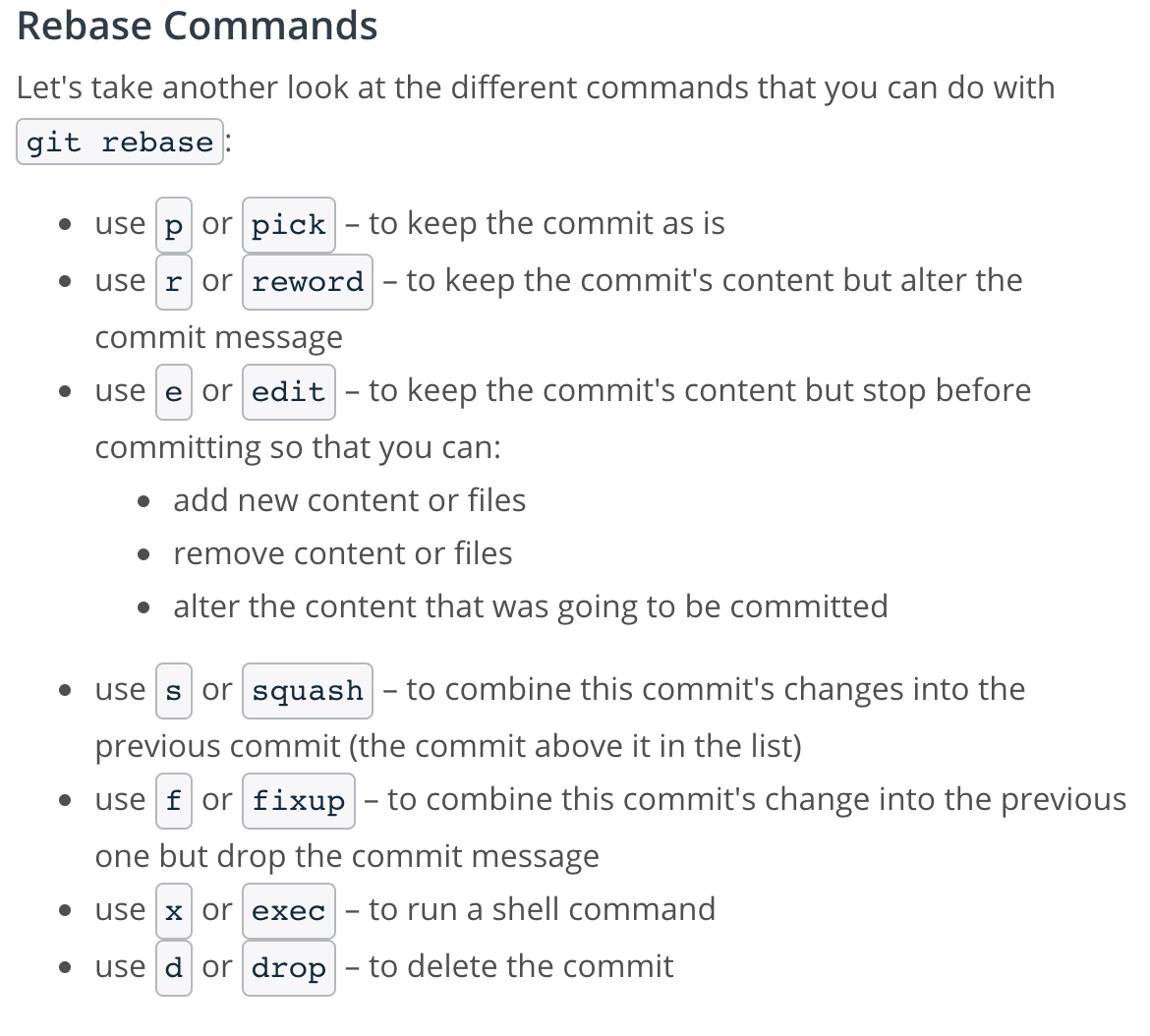